How to make API calls in Python using CLI
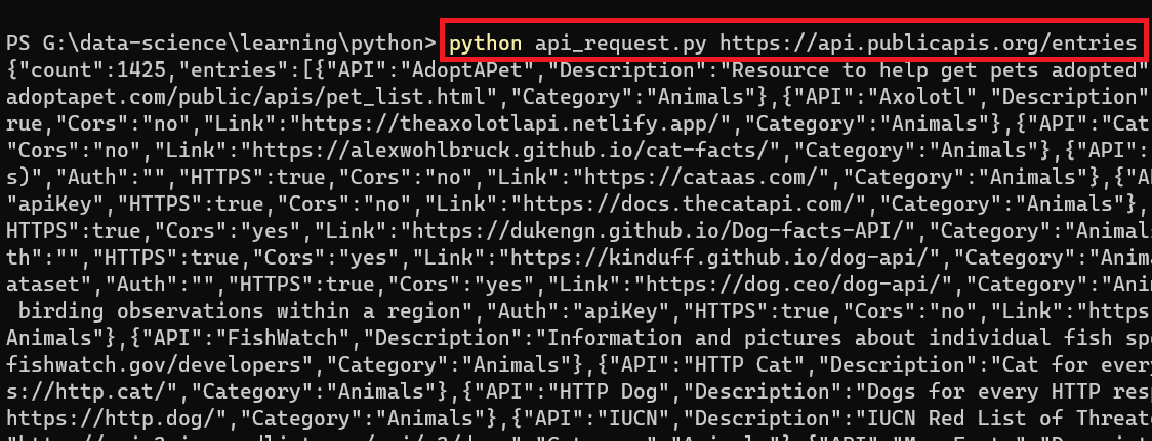
You can use the requests library in Python to make API requests, and you can create a script that accepts command-line arguments to specify the API URL.
1. Install the requests library if you haven't already.
2. Create a file named api_request.py
3. Copy/Paste below code in api_request.py
import argparse
import requests
def hit_api(api_url):
try:
response = requests.get(api_url)
response.raise_for_status() # Raise an exception for HTTP errors
return response.text
except requests.exceptions.RequestException as e:
return f"An error occurred: {e}"
if __name__ == "__main__":
parser = argparse.ArgumentParser(description="Hit an API using Python.")
parser.add_argument("url", help="URL of the API to hit")
args = parser.parse_args()
api_url = args.url
response_data = hit_api(api_url)
print(response_data)
4. Open a terminal and navigate to the directory containing the api_request.py file.
5. Run the script with the API URL as an argument.
python api_request.py https://api.publicapis.org/entries
This script uses the argparse library to handle command-line arguments and the requests library to make the API request. It prints the response data from the API or an error message if the request fails.
You can extend this script by adding more features, such as handling different HTTP methods (e.g., POST, PUT), passing headers or request payloads, and handling authentication if required by the API.
If you like what you are reading, please consider buying us a coffee ( or 2 ) as a token of appreciation.
Don't forget to share this article! Help us spread the word by clicking the share button below.
We appreciate your support and are committed to providing you valuable and informative content.
We are thankful for your never ending support.